I want the user to be asked 'how many circles' they wanna write until the user decides to end it with (Ctrl+d) which is EOF?
- C++ While Loop Example Program
- Flag Controlled While Loop C++
- Eof Controlled While Loop C++ Loop
- C++ While Not Eof
While input data file has correct data items, the program will read the data, and sum up the values, and record the number of items read until the EOF is encountered. You can use the pico or vi text editor to create the input data file according to the requirements of. Solution for What type of while loop is controlled with an input file counter-controlled while loop sentinel-controlled while loop O flag-controlled while loop.
extra question: if I write a letter for example 'k' it will spam out circles. How do I change that?
The biggest problem with your program is that scanf
will not read an EOF
into a variable. However, fixing just this problem is not going to make your program entirely correct, because there are other issues in your code:
- Your code repeats itself - when possible, you should unify the code that deals with the first iteration vs. subsequent iterations.
- Your code will not handle invalid input - when an end-user enters non-numeric data, your program goes into an infinite loop.
- Your code follows the old style of C - declaring all variables at the top has not been required for more than fifteen years. You should declare your loop variable inside the loop.
Here is how you fix all these shortcomings:
Array breaking in Pebble C
c,arrays,pebble-watch,cloudpebble
The problem is this line static char *die_label = 'D'; That points die_label to a region of memory that a) should not be written to, and b) only has space for two characters, the D and the 0 terminator. So the strcat is writing into memory that it shouldn't be..
Is it safe to read and write on an array of 32 bit data byte by byte?
c,memory,memory-alignment
Yes, this is correct. The only danger would be generating a bit pattern that does not correspond to any int, but on modern systems there are no such patterns. Also, if the data type was uint32_t specifically, those are prohibited from having any such patterns anyway. Note that the inverse..
Looping distinct values from one table through another without a join
sql,sql-server,tsql,while-loop
So you want all distinct records from table1 paired with all records in table2? That is a cross join: select * from (select distinct * from table1) t1 cross join table2; Or do you want them related by date? Then inner-join: select * from (select distinct * from table1) t1..
getchar() not working in c
c,while-loop,char,scanf,getchar
That's because scanf() left the trailing newline in input. I suggest replacing this: ch = getchar(); With: scanf(' %c', &ch); Note the leading space in the format string. It is needed to force scanf() to ignore every whitespace character until a non-whitespace is read. This is generally more robust than..
Counting bytes received by posix read()
c,function,serial-port,posix
Yes, temp_uart_count will contain the actual number of bytes read, and obviously that number will be smaller or equal to the number of elements of temp_uart_data. If you get 0, it means that the end of file (or an equivalent condition) has been reached and there is nothing else to..
Nested foreach loop in a While loop can make the condition for the while loop go over?
php,loops,foreach,while-loop
Glad you found an answer. Here is something I was working on while you found it. // SET START DATE $startDate = new DateTime($dateStart); $nextBill = new DateTime(); for($i=1;$i<$countDays;$i++){ switch(true){ case ($startDate->format('j') > $days[2]): // go to next month $nextBill->setDate( $startDate->format('Y'), $startDate->format('m')+1, $days[0]; ); break; case ($startDate->format('j') > $days[1]): //..
Segmentation fault with generating an RSA and saving in ASN.1/DER?
c,openssl,cryptography,rsa
pub_l = malloc(sizeof(pub_l)); is simply not needed. Nor is priv_l = malloc(sizeof(priv_l));. Remove them both from your function. You should be populating your out-parameters; instead you're throwing out the caller's provided addresses to populate and (a) populating your own, then (b) leaking the memory you just allocated. The result is..
C language, vector of struct, miss something?
c,vector,struct
What is happening is that tPeca pecaJogo[tam]; is a local variable, and as such the whole array is allocated in the stack frame of the function, which means that it will be deallocated along with the stack frame where the function it self is loaded. The reason it's working is..
Is there Predefined-Macros define about byte order in armcc
c,armcc,predefined-macro
Well according to this page: http://www.keil.com/support/man/docs/armccref/armccref_BABJFEFG.htm You have __BIG_ENDIAN which is defined when compiling for a big endian target..
How to control C Macro Precedence
c,macros
You can redirect the JOIN operation to another macro, which then does the actual pasting, in order to enforce expansion of its arguments: #define VAL1CHK 20 #define NUM 1 #define JOIN1(A, B, C) A##B##C #define JOIN(A, B, C) JOIN1(A, B, C) int x = JOIN(VAL,NUM,CHK); This technique is often used..
Set precision dynamically using sprintf

c,printf,format-string
Yes, you can do that. You need to use an asterisk * as the field width and .* as the precision. Then, you need to supply the arguments carrying the values. Something like sprintf(myNumber,'%*.*lf',A,B,a); Note: A and B need to be type int. From the C11 standard, chapter §7.21.6.1, fprintf()..
Disadvantages of calling realloc in a loop
c,memory-management,out-of-memory,realloc
When you allocate/deallocate memory many times, it may create fragmentation in the memory and you may not get big contiguous chunk of the memory. When you do a realloc, some extra memory may be needed for a short period of time to move the data. If your algorithm does..
Updating jTextArea in while loop
java,swing,while-loop,jtextarea,updating
Correction: This won't help since the infinite loop will still block the EDT forever.. Nevermind! Your while loop is a really bad idea, but if you insist, you can at least give the EDT a chance to update the UI by dispatching your append asynchronously: SwingUtilities.invokeLater(new Runnable() { @Override public..
How convert unsigned int to unsigned char array
c++,c
#include <stdio.h> int main() { unsigned int i = 0x557e89f3; unsigned char c[4]; c[0] = i & 0xFF; c[1] = (i>>8) & 0xFF; c[2] = (i>>16) & 0xFF; c[3] = (i>>24) & 0xFF; printf('c[0] = %x n', c[0]); printf('c[1] = %x n', c[1]); printf('c[2] = %x n', c[2]); printf('c[3] =..
C programming - Confusion regarding curly braces
c,scope
The only difference between the two is the scope of the else. Without the braces, it spans until the end of the full statement, which is the next ;, i.e the next line: else putchar(ch); /* end of else */ lastch = ch; /* outside of if-else */ With the..
Text justification C language
c,text,alignment
From printf's manual: The field width An optional decimal digit string (with nonzero first digit) specifying a minimum field width. If the converted value has fewer characters than the field width, it will be padded with spaces on the left (or right, if the left-adjustment flag has been given). Instead..
Program to reverse a string in C without declaring a char[]
c,string,pointers,char
Important: scanf(' %s', name); has no bounds checking on the input. If someone enters more than 255 characters into your program, it may give undefined behaviour. Now, you have the char array you have the count (number of char in the array), why do you need to bother doing stuffs..
How to increment the value of an unsigned char * (C)
c++,c,openssl,byte,sha1
I am assuming your pointer refers to 20 bytes, for the 160 bit value. (An alternative may be text characters representing hex values for the same 160 bit meaning, but occupying more characters) You can declare a class for the data, and implement a method to increment the low order..
How are the results for count different in all these three cases?
python,for-loop,while-loop,break
For Code 1, you're continuing to add on to the count. So during the first iteration, the count becomes 12 (the length of 'hello, world' is 12), and then during the second iteration, you never reset the count to 0 so count is continuously added on until it reaches 24,..
Galois LFSR - how to specify the output bit number
c,prng,shift-register
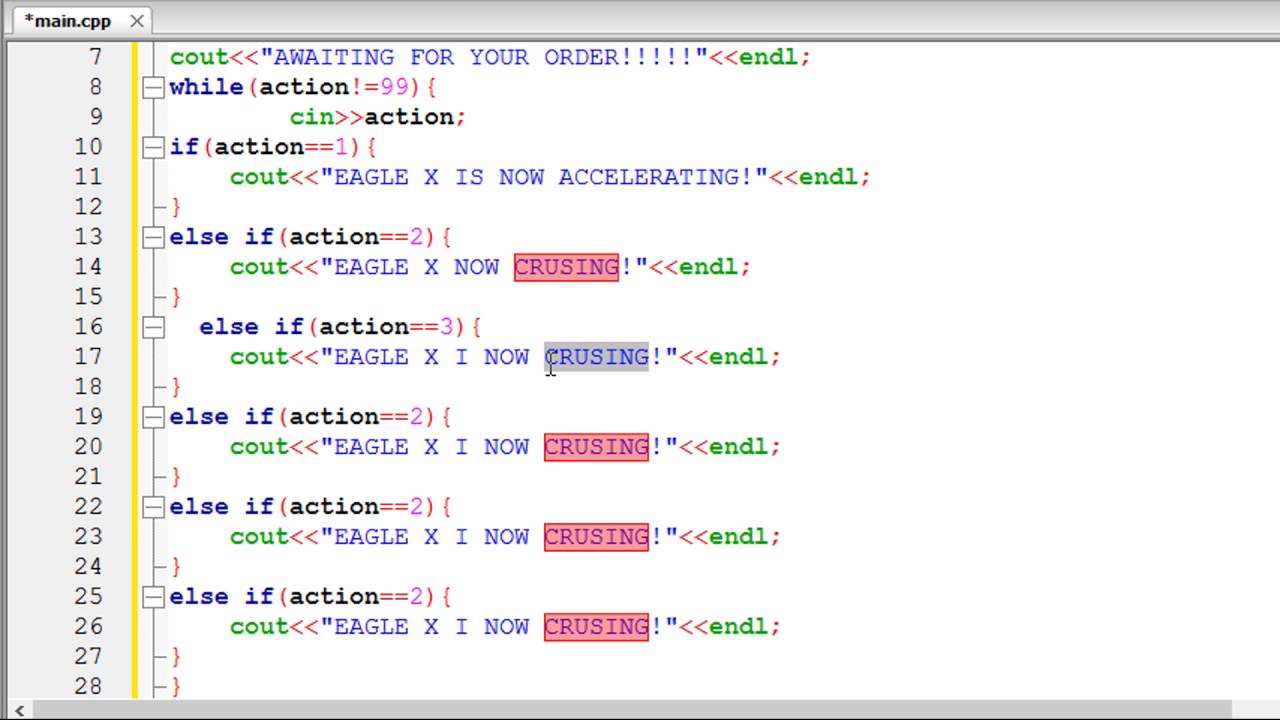
If you need bit k (k = 0 .15), you can do the following: return (lfsr >> k) & 1; This shifts the register kbit positions to the right and masks the least significant bit..
CGO converting Xlib XEvent struct to byte array?
c,go,xlib,cgo
As mentioned in the cgo documentation: As Go doesn't have support for C's union type in the general case, C's union types are represented as a Go byte array with the same length. Another SO question: Golang CGo: converting union field to Go type or a go-nuts mailing list post..
How does this code print odd and even?
c,if-statement,macros,logic
In binary any numbers LSB (Least Significant Bit) is set or 1 means the number is odd, and LSB 0 means the number is even. Lets take a look: Decimal binary 1 001 (odd) 2 010 (even) 3 011 (odd) 4 100 (even) 5 101 (odd) SO, the following line..
What all local variables goto Data/BSS segment?
c++,c,nm
'local' in this context means file scope. That is: static int local_data = 1; /* initialised local data */ static int local_bss; /* uninitialised local bss */ int global_data = 1; /* initialised global data */ int global_bss; /* uninitialised global bss */ void main (void) { // Some code..
How to read string until two consecutive spaces?
c,format,sscanf,c-strings
The scanf family of functions are good for simple parsing, but not for more complicated things like you seem to do. You could probably solve it by using e.g. strstr to find the comment starter '//', terminate the string there, and then remove trailing space..
Efficient comparison of small integer vectors
c,integer,compare,bit-manipulation,string-comparison
It's possible to do this using bit-manipulation. Space your values out so that each takes up 5 bits, with 4 bits for the value and an empty 0 in the most significant position as a kind of spacing bit. Placing a spacing bit between each value stops borrows/carries from propagating..
How can I align stack to the end of SRAM?
c,embedded,stm32,gnu-arm,coocox
I've found the reason: that's because stack size is actually fixed and it is located in heap (if I could call it heap). In file startup_stm32f10x*.c there is a section: /*----------Stack Configuration----------*/ #define STACK_SIZE 0x00000100 /*!< The Stack size suggest using even number */ And at then very next line:..
What does `strcpy(x+1, SEQX)` do?
c,strcpy
The pointer + offset notation is used as a convenient means to reference memory locations. In your case, the pointer is provided by malloc() after allocating sufficient heap memory, and represents an array of M + 2 elements of type char, thus the notation as used in your code represents..
Segmentation Fault if I don't say int i=0
c,arrays,segmentation-fault,initialization,int
In your code, int i is an automatic local variable. If not initialized explicitly, the value held by that variable in indeterministic. So, without explicit initialization, using (reading the value of ) i in any form, like array[i] invokes undefined behaviour, the side-effect being a segmentation fault. Isn't it automatically..
VS2012 Identifer not found when part of static lib
c,visual-studio-2012,linker,static-libraries
C++ uses something called name mangling when it creates symbol names. It's needed because the symbol names must contain the complete function signature. When you use extern 'C' the names will not be mangled, and can be used from other programming languages, like C. You clearly make the shunt library..
Reverse ^ operator for decryption
c,algorithm,security,math,encryption
This is not a power operator. It is the XOR operator. The thing that you notice for the XOR operator is that x ^ k ^ k x. That means that your encryption function is already the decryption function when called with the same key and the ciphertext instead..
free causing different results from malloc
c,string,malloc,free
Every time you are creating your string, you are not appending a null terminator, which causes the error. So change this: for(j=0; j<rem_len; j++) { if(j != i) { remaining_for_next[index_4_next] = remaining[j]; index_4_next++; } } to this: for(j=0; j<rem_len; j++) { if(j != i) { remaining_for_next[index_4_next] = remaining[j]; index_4_next++; }..
OpenGL glTexImage2D memory issue
c,opengl
Which man page are you quoting? There are multiple man pages available, not all mapping to the same OpenGL version. Anyways, the idea behind the + 2 (border) is to have 2 multiplied by the value of border, which is in your case 0. So your code is just fine..
Does strlen() always correctly report the number of char's in a pointer initialized string?
c,strlen
What strlen does is basically count all bytes until it hits a zero-byte, the so-called null-terminator, character '0'. So as long as the string contains a terminator within the bounds of the memory allocated for the string, strlen will correctly return the number of char in the string. Note that..
Is post-increment operator guaranteed to run instantly?
c,c89,post-increment,ansi-c
This code is broken for two reasons: Accessing a variable twice between sequence points, for other purposes than to determine which value to store, is undefined behavior. There are no sequence points between the evaluation of function parameters. Meaning anything could happen, your program might crash & burn (or more..
C++ / C #define macro calculation
c++,c,macros
Are DETUNE1 and DETUNE2 calculated every time it is called? Very unlikely. Because you are calling sqrt with constants, most compilers would optimize the call to the sqrt functions and replace it with a constant value. GCC does that at -O1. So does clang. (See live). In the general..
Java Scanner not reading newLine after wrong input in datatype verification while loop
java,while-loop,java.util.scanner
You are reading too much from the scanner! In this line while (sc.nextLine() ' || sc.nextLine().isEmpty()) you are basically reading a line from the scanner, comparing it (*) with ', then forgetting it, because you read the next line again. So if the first read line really contains the..
CallXXXMethod undefined using JNI in C
java,c,jni
There are few fixes required in the code: CallIntMethod should be (*env)->CallIntMethod class Test should be public Invocation should be jint age = (*env)->CallIntMethod(env, mod_obj, mid, NULL); Note that you need class name to call a static function but an object to call a method. (cls2 -> person) mid =..
How does ((a++,b)) work? [duplicate]
c,function,recursion,comma
In your first code, Case 1: return reverse(i++); will cause stack overflow as the value of unchanged i will be used as the function argument (as the effect of post increment will be sequenced after the function call), and then i will be increased. So, it is basically calling the..
Pygame nested while loop: [Errno 10054] An existing connection was forcibly closed by the remote host
C++ While Loop Example Program
python,while-loop,pygame
You need to call pygame.event.pump() inside your inner while loop to ensures that your program can internally interact with the rest of the operating system. # .. while a: pygame.event.pump() keys2 = pygame.key.get_pressed() # do something .. An alternative would be to listen for pygame.KEYDOWN events on the event queue..
Is i=i+1 an undefined behaviour?
c,increment,undefined-behavior
There is no undefined behavior in this code. i=i+1; is well-defined behavior, not to be confused with i=i++; which gives undefined behavior. The only thing that could cause different outputs here would be floating point inaccuracy. Try value += 4 * (int)nearbyint(pow(10,i)); and see if it makes any difference..
execl() works on one of my code, but doesn't work on another
c,execl
My C is a bit rusty but your code made many rookie mistakes. execl will replace the current process if it succeeds. So the last line ('i have no idea why') won't print if the child can launch successfully. Which means.. execl failed and you didn't check for it! Hint:..
C binary tree sort - extending it
c,binary-tree,binary-search-tree
a sample to modify like as void inorder ( struct btreenode *, int ** ) ; int* sort(int *array, int arr_size) { struct btreenode *bt = NULL; int i, *p = array; for ( i = 0 ; i < arr_size ; i++ ) insert ( &bt, array[i] ) ;..
scanf get multiple values at once
c,char,segmentation-fault,user-input,scanf
I'm not saying that it cannot be done using scanf(), but IMHO, that's not the best way to do it. Instead, use fgets() to read the whole like, use strtok() to tokenize the input and then, based on the first token value, iterate over the input string as required. A..
Does realloc() invalidate all pointers?
c,pointers,dynamic-memory-allocation,behavior,realloc

Yes, ptr2 is unaffected by realloc(), it has no connection to realloc() call whatsoever(as per the current code). However, FWIW, as per the man page of realloc(), (emphasis mine) The realloc() function returns a pointer to the newly allocated memory, which is suitably aligned for any kind of variable and..
Loop through database table and compare user input
mysql,c
If you are only looking for fields that match the input, you'll want to search the database using the input string. In other words, write your query string so that it only gives you results that match the user input. This will be much faster than searching through every returned..
Infinite loop with fread
c,arrays,loops,malloc,fread
I just found out how to make rainbow sparkles cursor stuff and now I’m happy. I mean, LOOK AT IT GO:DDDDD. #sparkle cursor #local newbie. I finally have those cursors that drop sparkles when you move your mouse on my tumblr and im- 🥺 i got the code from a website called snazzyspace:) #sparkle cursor #miscellaneous. Find and follow posts tagged sparkle cursor on Tumblr. Tutorial: sparkle cursor. I finally have those cursors that drop sparkles when you move your mouse on my tumblr and im- 梁 i got the code from a website called snazzyspace:) #sparkle cursor #miscellaneous. Tumblr mouse sparkles. Please don't forget to check our NOTICES tab on the right, and do check out the COMMONLY ASKED and BASIC CODES sections, a lot of your answers are there. We are a blog designed to provide tutorials that will teach you how to add cool features to your blog, as well as answer any questions you have about coding/html/editing your theme:). Tumblr Mouse Sparkle Trails This tumblr code will put sparkles to follow your mouse cursor! Just paste the following code right before the part of your HTML under the customize section in Tumblr. Below are several colours to choose from, but if you're advanced at codes you can change the color yourself in the code! . View your page and you should have pretty rainbow mouse sparkles:). With html codes. You can find everything for your tumblr here from themes & tutorials to cursors & fonts. I try my best to keep the site updated, but am currently working full time so will update when i can.
If you're 'trying to allocate an array 64 bytes in size', you may consider uint8_t Buffer[64]; instead of uint8_t *Buffer[64]; (the latter is an array of 64 pointers to byte) After doing this, you will have no need in malloc as your structure with a 64 bytes array inside is..
fread(), solaris to unix portability and use of uninitialised values
c,linux,memory,stack,portability
Q 1. why is ch empty even after fread() assignment? (Most probably) because fread() failed. See the detailed answer below. Q 2.Is this a portability issue between Solaris and Linux? No, there is a possible issue with your code itself, which is correctly reported by valgrind. I cannot quite..
Passing int using char pointer in C
c,exec,ipc
Programs simply do not take integers as arguments, they take strings. Those strings can be decimal representations of integers, but they are still strings. So you are asking how to do something that simply doesn't make any sense. Twenty is an integer. It's the number of things you have if..
My While True loop is getting stuck in python
python,while-loop,raspberry-pi,infinite-loop,raspbian
You need to keep updating your input_* variables inside your while loop while True: input_A = GPIO.input(26) input_B = GPIO.input(19) input_C = GPIO.input(13) input_D = GPIO.input(6) if input_A True: print('A was pushed') if input_B True: print('B was pushed') if input_C True: print('C was pushed') if input_D ..
- Related Questions & Answers
- Selected Reading
EOF
EOF stands for End of File. The function getc() returns EOF, on success.
Here is an example of EOF in C language,
Let’s say we have “new.txt” file with the following content.
Now, let us see the example.
Example
Output
In the above program, file is opened by using fopen(). When integer variable c is not equal to EOF, it will read the file.
getc()
It reads a single character from the input and return an integer value. If it fails, it returns EOF.
Here is the syntax of getc() in C language,
Here is an example of getc() in C language,
Let’s say we have “new.txt” file with the following content −
Now, let us see the example.
Example
Output
In the above program, file is opened by using fopen(). When integer variable c is not equal to EOF, it will read the file. The function getc() is reading the characters from the file.
feof()
The function feof() is used to check the end of file after EOF. Parallels desktop 11 serial key mac. It tests the end of file indicator. It returns non-zero value if successful otherwise, zero.
Here is the syntax of feof() in C language,
Here is an example of feof() in C language,
Flag Controlled While Loop C++
Let’s say we have “new.txt” file with the following content −
Now, let us see the example.
Eof Controlled While Loop C++ Loop
Example
Output
C++ While Not Eof
In the above program, In the above program, file is opened by using fopen(). When integer variable c is not equal to EOF, it will read the file. The function feof() is checking again that pointer has reached to the end of file or not.
